How to Test HTTP Responses and Redirects with Cypress
All sites have different Http Codes like 200, 404, 500, 300 and we can test them easily to always send back the right response!
By Riccardo Giorato in cypress e2e http-status
Published on Tue Sep 28 2021
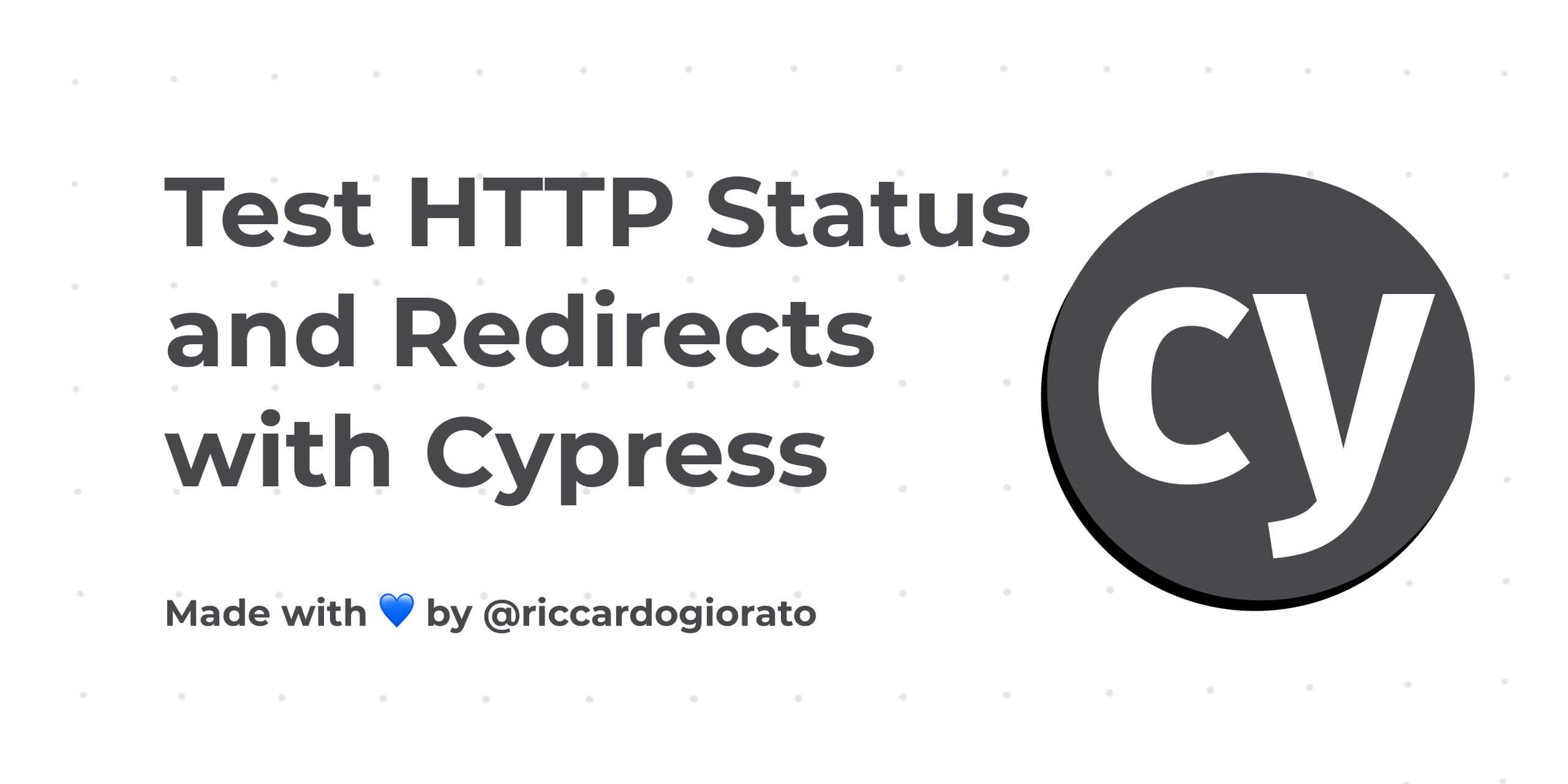
All the code from this tutorial here: https://github.com/riccardogiorato/cypress-for-everything/tree/main/examples/http-response-status
Why making an E2E test for a Http Responses?
The under users of your app will always visit your website from specific urls or maybe in many occasions they will make a spell error in the URL maybe forgetting the “s” in “https://” or not adding the “www” to the URLs.
With Cypress you can test all sort of these things usually done with 300 or 301 redirects from the Servers or also testing responses to 404 pages or 500 forbidden pages from unauthenticated users.
For this tutorial, we choose to use Cypress.io cause it’s one of the most used E2E tools on the web.
Redirects 301 code
Redirects usually are done with the code 301 “Moved Permanently”, they are used when you a specific page or url has been reorganized or moved to a different one.
const baseUrlTesla = "https://www.tesla.com/"
const urlHttp = "http://tesla.com"
it(urlHttp + " end location", () => {
cy.visit(urlHttp)
cy.url().should("eq", baseUrlTesla)
})
it(urlHttp + " redirect", () => {
cy.request({
url: urlHttp,
followRedirect: false, // turn off following redirects
}).then((resp) => {
// redirect status code is 301
expect(resp.status).to.eq(301)
expect(resp.redirectedToUrl).to.eq(baseUrlTesla)
})
})
Found Page 200 code
The “200” response code is used for all found pages, when the server exactly finds the resource at the URL you specified in your request.
const baseUrlTesla = "https://www.tesla.com/"
const urlHttpsWww = "https://www.tesla.com/"
it(urlHttpsWww + " end location", () => {
cy.visit(urlHttpsWww)
cy.url().should("eq", baseUrlTesla)
})
it("200 homepage response", () => {
cy.request({
url: urlHttpsWww,
followRedirect: false,
}).then((resp) => {
expect(resp.status).to.eq(200)
expect(resp.redirectedToUrl).to.eq(undefined)
})
})
Not Found Page 404 code
When you don’t find a page you will get the most beautiful and common code 404 also know “not found”!
const baseUrlTesla = "https://www.tesla.com/"
const url404test = "https://www.tesla.com/not-a-real-page"
it("404 'not found' response", () => {
cy.request({
url: url404test,
followRedirect: false,
failOnStatusCode: false,
}).then((resp) => {
expect(resp.status).to.eq(404)
expect(resp.redirectedToUrl).to.eq(undefined)
})
cy.visit(url404test, { failOnStatusCode: false })
cy.get(".error-code").should("contain", "404")
cy.get(".error-text").should("contain", "Page not found")
})
Conclusion
There is no right or wrong way to build an E2E test. The only thing you should care about is building a proper test that will automate your manual actions.
With this tutorial, we won’t ever need to check again the usual pages we have for 404, we will always be able to check all the redirects we implemented and more!
Less time to do a manual test and more time to have fun building other things!
Let us know in the comments which kind of test you would like to see next!
Resources
Testing Tesla Http Responses: https://github.com/riccardogiorato/cypress-for-everything/blob/main/examples/http-response-status/cypress/integration/tesla-http.ts
Cypress Example directory: cypress-for-everything#examples
Http Response examples: https://github.com/riccardogiorato/cypress-for-everything/tree/main/examples/http-response-status